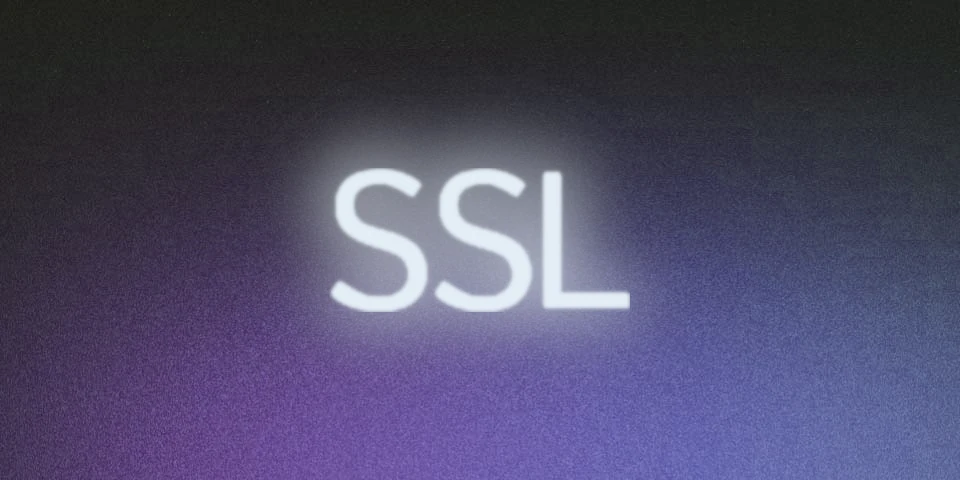
Simple Scripting Language
Simple Scripting Language was the first programming language I made and set the stage for my work on Tempered.
let foo = "foo"
let bar = if foo == "foo" {
true
} else {
false
}
print bar
>>> true
For this project, I implemented a lexer, paser and interpreter. There’s a great slide from an Andrew Kelly talk that explained this best
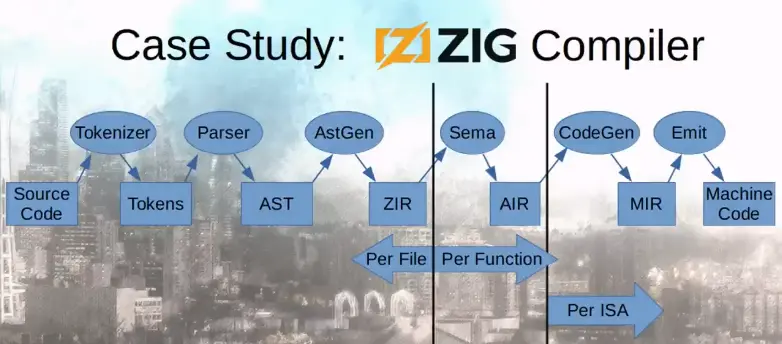
Each step in a compiler is taking an input data structure (text, tokens, AST) and converting it into the next stage’s data structure.
For this project, I only implemented a Tokenizer/Lexer, Parser and Interpreter.
In a more complicated interpreter, you’d compile the source code into byte code. This is a low level, but still platform independant version of your code. By using bytecode, you can read instructions in from an array which is much faster due to being really efficent to read from memory.
However, I didn’t do that for this project. Instead I wrote a really simple tree walking interpreter. It walks over the syntax tree, evaluating expressions as it went and executing statements. Assignment works by just storing everything in a table and referencing that. Printing just logs out the variable in a different way depending on the type, and if statements simply check if the value is